If you’re gearing up for a tech interview, chances are Java 8 interview questions will pop up – and not just because your interviewer wants to relive 2014, but because the language is now popular once again.
Despite being nearly a decade old, Java 8 remains a favorite in the programming world, powering 30% of global developer activity as of 2024.
With features like Lambda expressions, Stream API, and Optional in Java 8, it’s no surprise that Java ranks among the top three most in-demand programming languages, alongside Python and JavaScript.
Coming back to the question – Why does Java 8 still dominate? It’s because enterprises love stability, security, and scalability—and Java delivers all three. From backend systems to Android apps that run on 83% of global devices, Java is a Swiss Army knife for developers.
Now, imagine tackling interview questions that tap into all this versatility. This article is your roadmap to acing those questions, blending humor and expertise to turn complex topics into clear wins. Ready? Let’s dive in.
Java 8 Interview Questions for Freshers
1.What is Java 8?
The very first Java 8 interview question you may come across is this one right here. As easy as this question may sound, many still fail to provide the employer with a perfect answer.
Java 8 is an advanced version of the traditional Java programming language and platform, launched in March 2014. Java 8 modernized traditional Java, making it more efficient and developer-friendly.
Several revolutionary changes came with it, changing how developers can write code. Some important updates are lambda expressions, which make the code more compact, and the Stream API, which has made the processing of the code convenient.
2.Why are Lambda Expressions important?
The most significant improvements in Java 8 come with Lambda Expressions, which are the introduction to functional programming.
Before introducing Lambda Expressions, sorting and similar operations in Java often required implementations of the `Comparator` interface, which was verbose. With Lambdas, developers can write shorter and more readable code without a boilerplate.
So, while answering your Java 8 coding interview questions, you need to highlight that it helps developers save much more time during development and get cleaner, easier-to-maintain code. Lambdas are especially useful together with the Stream API since they allow filtering, mapping, and reducing collections in just a few lines of code.
3.What Is The Purpose of Stream API?
The Stream API was created to process collections of data in a functional and declarative way.
A pipeline of operations is introduced, through which one can filter, map, sort, and reduce data without changing the original collection.
This makes the Java code more declarative and easier to comprehend. In contrast, it could significantly boost performance on multi-core processors.
The Stream API is also lazy. Hence, operations are executed only when a terminal method, like `collect` or `forEach,` is invoked for further performance enhancement.
While preparing for your Java 8 coding interview questions, you should know the answer to this question!
4.What are Default Methods?
This is one of the most common Java 8 coding interview questions! The answer is that default methods allow developers to add new functionality to interfaces without breaking existing implementations. Before Java 8, modifying an interface required changes in all classes that implemented it, which could lead to extensive refactoring. Default methods solve this by providing a default implementation directly in the interface.
For example, suppose a new method is added to a broadly used interface: developers can provide a default method to ensure backend compatibility. It makes evolving APIs while preserving older code bases much easier. Moreover, it makes multiple inheritance of behavior possible by allowing classes to implement several interfaces with overlapping default methods.
5.What does the Optional class represent?
A long-standing problem with null pointer exceptions has plagued Java developers for decades; Java 8 corrects this by introducing the Optional class – which is a container that may or may not hold a non-null value.
Rather than returning `null` directly, methods can be designed to return an `Optional` object, and developers are then forced to check explicitly for the absence of a value using methods such as `present,` `orElse,` or `ifPresent.`
As an example, a block of code can be executed safely only when the value is not null. This helps one avoid checking for null, results in fewer bugs and encourages clean and more readable code through better design.
While preparing for Java 8 interview questions, you should know this one!
- Struggling to keep up with Java 8’s game-changing features?
- Tekrevol’s expert training resources can help you master Java 8 and prepare for interviews with confidence.
Java 8 Features Interview Questions
6.List some of the key features of Java 8
While preparing for the Java 8 features interview questions, this one is surely the most important!
Java 8 introduced some groundbreaking features that modernized the language, making it more efficient and expressive. One of the renowned features is “Lambda Expressions,” which allows you to write very concise functional code, and the Stream API, which allows you to do bulk operations for making collections, filtering, or mapping.
Other than that, the “default Methods” were introduced to the interfaces to allow backend compatibility as new methods were added. Not only that, the “Optional” feature was introduced to reduce null pointer exceptions by providing a safer means of handling nulls.
7.What are Functional Interfaces?
A functional Interface in Java 8 consists of a single abstract method, making it well-suited for use with Lambda Expressions. This is based on the functional programming paradigm, where functions may be passed as arguments. Some more examples are `Runnable,` `Callable,` and `Comparator.` Starting from Java 8, an annotation `@FunctionalInterface` has been added to enforce this constraint.
This documentation will prevent the addition of any abstract methods that would change its functionality in some way. Functional Interfaces support concise coding by providing the basis for using brief, anonymous functions.
Since this Java 8 interview question focuses on the foundation of the language, you should know the answer to this!
8.Describe the importance of the Stream API.
One of Java 8’s most revolutionary features is the Stream API, which allows developers to perform functional-style data processing. Streams provide developers with a far more declarative way to talk about collections— a little less about what to do and much more about what to achieve.
For instance, filtering, mapping, and reducing collections can all be done super easily. Intermediate operations, such as `filter,` are only executed upon invocation of a terminal operation, like `collect.`
This makes the process run much better as data are processed based on demand. Streams also support parallel data processing, using all available cores for computational efforts. This produces clean, efficient, and maintainable code.
9.How does the new Date and Time API work?
The Date and Time API in Java 8 fixes the problems of the old `Date` and `Calendar` classes, such as thread safety issues and poor design. The new API introduces immutable and thread-safe classes like `LocalDate,` `LocalTime,` and `LocalDateTime,` which represent dates and times without relying on system-specific zones.
In these classes, manipulation works by the builder style, so adding days or formatting operations is made easier. There is also `ZonedDateTime` in the `java. Time` package for handling time zones and `Duration` for time intervals. This new API makes for much smoother date handling in Java in terms of readability, correctness, and ease of use.
This one is for sure the most asked Java 8 Interview question that we’ve come so far!
10.What is the role of Nashorn in Java 8?
Nashorn is a lightweight JavaScript engine introduced in Java 8. It enables developers to execute JavaScript code within a Java application. It replaces the older Rhino engine and offers improved performance by leveraging the Java Virtual Machine (JVM).
Nashorn supports the standards of ECMA script; hence, this makes it perfect for embedding scripts within the Java application or interacting dynamically with web components. The developers can actually run JavaScript code from the command line using the tool `js.` Though Nashorn has been obsolete in the later releases of Java, it remains a remarkable addition to Java 8.
While preparing for Java 8 coding interview questions, this one shouldn’t be missed!
Important Questions on Java 8 for Experienced Candidates
11.How does Java 8 help support functional programming?
Java 8 introduced features similar to functional programming ideas, such as “expressions, Stream API, and Functional Interfaces.”
Functional programming emphasizes immutability, declarative code, and treating functions like first-class citizens. Lambda Expressions represent the writing of compact, anonymous methods that can be treated as arguments. However, the new addition of the Stream API in Java 8 lets us describe operations over collections, such as filtering and mapping, in a declarative way.
Functional Interfaces, such as `Predicate` and `Function,` form the cornerstone of functional programming. Such additions empower Java to undertake a programming paradigm with fewer side effects, more readability, and more modern code development.
12.What is method reference in Java 8?
A method reference is a short notation to refer to a method by its name instead of writing it explicitly in a Lambda Expression. It increases readability by removing redundancy if the Lambda body just calls an existing method. There are four types of method references:
Static methods: `ClassName::staticMethod`
Instance methods of a specific object: `instance::instanceMethod.`
Instance methods of any object: `ClassName::instanceMethod.`
Constructor references: `ClassName::new.`
While preparing for the Java 8 coding interview questions, you can be asked for an example for method reference. Here’s one for you!
For instance, instead of writing `(x) -> System. Out. println(x)`, you use `System.out::println`. It makes the code cleaner and more compact, and thereby closer to the goals of the Java functional programming initiative.
13.What is the difference between Collection and Stream API?
The Collection API focuses on operations related to storing data in memory using simple methods like `add,` `remove,` and `get.` It is mainly used for storing data and reading it out. On the contrary, the Stream API focuses on processing data by applying operations in a functional style. Streams allow for filtering, mapping, and reducing without the use of any explicit modification of the underlying data structure.
In Java 8 coding interview questions, the difference between Collection and Stream API often confuses the developers, so you should remember the answer to this question here!
14.Explain the use of `forEach` in Java 8.
In Java 8, the `forEach` method can be used to iterate elements in a collection or stream. It is non-executable and requires that you pass it a Lambda Expression or method reference that will perform actions on each of the elements. For instance, you can use it to print, modify, or process data.
Unlike traditional loops, `forEach` is declarative, reducing boilerplate and improving readability. In Streams, it’s a terminal operation, meaning the Stream cannot be reused after `forEach` has been executed. However, be careful: for stateful operations (such as modifying external variables), avoid overusing `forEach,` which might yield unexpected results, especially with parallel streams.
15.How does Java 8 handle backend compatibility?
Java has always been a technology that emphasizes backend compatibility, and Java 8 maintains it by providing features such as “Default Methods” in interfaces. The developer can add new functionality to an interface without breaking existing implementations with default methods.
For instance, when a new method is added to an interface, older classes that implement it can still keep working and do not necessarily require any change as they automatically inherit the default implementation.
But the magic does not stop there. The most fundamental changes in Java 8, like the Stream API and Lambda Expressions, do not break older code into pieces. The streams will even work on legacy collections. In other words, Java 8 balances innovation with stability: modern features without breaking their existing codebases.
Backend compatibility is often asked in Java 8 coding interview questions. Hence, you need to have a good grip on it.
- Struggling With Scenario-Based Coding Challenges?
- Learn from Tekrevol’s Industry-Leading Java Mentors and Elevate Your Problem-Solving Skills.
- Get Your Java Consultation Now!
Java 8 Stream API Interview Questions
16.What is the difference between `map` and `flatMap`?
Both map and flatMap are the methods used under the Stream API for transforming data, though they are different in purpose.
Map: It applies a function to each element of the stream and transforms it into a new stream. For example, transform a list of strings into a list of their lengths.
flatMap: this is where transformation results in a stream. This levels the nested streams into a single continuous stream; hence, there are no nested structures.
For anyone preparing for Java 8 interview questions, this one here is a must!
17.Is the Stream API lazy?
Yes, the Stream API is lazy by default; it creates computation until a terminal operation is executed. For example, operations such as `filter` or `map’ are intermediate operations. They are not run immediately but stored in a pipeline instead.
A pipeline of operations only runs until a terminal operation like `collect` or `forEach` is requested on it. This lazy behavior makes Streams efficient since it avoids processing data unnecessarily. For example, when filtering a huge dataset, only the required elements for the termination operation are processed, guaranteeing optimal performance.
18.What are intermediate and terminal operations in Streams?
Operations in the Stream API are classified into two types:
Intermediate operations: These return another stream and are lazy. One such example is “filters.” They are used to build a pipeline of processing and do not execute until the terminal operation is invoked.
Terminal Operations: They have a side effect and result and mark the start of the execution of the stream pipeline. Examples include `collect,` `forEach,` and `reduce.` Once the terminal operation is performed, the stream pipeline is consumed and cannot be reused.
The distinction between Stream’s operations must be on your to-do list while preparing for Java 8 coding interview questions.
19.Can Streams modify the source collection?
To track the candidate, the interviewer often loves asking about this Java 8 coding interview question! The answer to this is No; streams are not destructive and immutable; they do not change the source collection from which they were derived. Instead, streams produce a new sequence of information to process.
For instance, if you use a `filter` operation on a list, then the original list remains unchanged, and a new filtered stream is created. This is what ensures thread safety and makes streams optimal for concurrent programming.
In case you need to update the source, you can collect the stream’s results into a new collection and then update the source manually.
20.How is parallelStream different from Stream?
The only difference between `Stream` and `parallel stream` is in the processing of data.
Stream: This is a sequential way to process one element at a time in the order they appear. It is best suited for small data sets or when order matters.
ParallelStream: This approach splits the data into chunks and processes them in parallel using multiple threads. It leverages multi-core processors, making it suitable for large datasets or computationally intensive operations.
While `parallel stream` can significantly improve performance, it also introduces complexity, such as potential thread safety issues. It’s crucial to evaluate the use case before deciding to use parallel streams.
If you are passionate about making your career in tech, you need to pin this Java 8 coding interview question in your head!
Lambda Expression Interview Questions in Java
21.What are Lambda Expressions?
In Java 8 features interview questions, you are often asked what are the Lambda Expressions. Well, it’s a new feature in Java 8 that allows developers to write anonymous methods in a functional style.
These expressions enable concise and expressive syntax, eliminating the need for boilerplate code like anonymous inner classes. Lambdas are especially useful for tasks such as iterating through collections, sorting, filtering, or mapping data.
By adopting the functional programming principles, they make Java code modern and easier to read. For instance, where creating a separate class or interface implementation was demanded for simple work, Lambdas allows you to inline the functionality directly where needed, both in clarity and efficiency.
22.How do Lambdas simplify code?
Lambdas simplifies Java code by eliminating boilerplate and reducing verbosity. Before Java 8, implementing even a simple task required a lot of code: defining a class, implementing an interface, and overriding a method. Now, using Lambdas, this could be condensed to a single concise expression:
Instead of a fully written `Comparator` class, you might write `(a, b) -> a.compareTo(b).` That does make the code cleaner, more readable, and easier to maintain. The alignment to the principles of functional programming helps Java compete in terms of code brevity with Python and JavaScript.
While preparing for Java 8 features interview questions, you shouldn’t miss this one!
23.Do Lambda Expressions support parameter overloading?
Yes, Lambda Expressions support passing more than one argument. In the parentheses `(param1, param2 )`, you define them separately with a comma. For instance, if you want to perform some arithmetic on two integers, you can declare a Lambda Expression like `(x, y) -> x + y`.
The number of parameters should match the method signature of the functional interface being implemented. Lambdas also supports type inference, so you don’t need to declare the parameter types unless required for clarity explicitly. This flexibility makes Lambdas a versatile tool for concisely handling complex operations.
24.What is the syntax of a Lambda Expression?
The syntax of a Lambda Expression in Java is: `(parameters) -> { body }.` It is made up of three constituents:
Parameters: A list of arguments, which can be empty, single, or multiple, placed enclosed in parentheses. Types are optional because of type inference.
Arrow operator (`->`): This separates the parameters from the body.
Body: The block of code executed when the Lambda is invoked. For single-line bodies, curly braces are optional; for multiple lines, they’re mandatory.
For instance, `(x, y) -> x + y` is a simple Lambda that adds two numbers. This syntax helps write logic in line without defining separate methods. Remembering syntax is a must when you are preparing for Java 8 features interview questions!
25.Where can Lambda Expressions be used?
Lambda expressions can be used anywhere a Functional Interface is needed. Functional interfaces have at most one abstract method such as include `Runnable,` `Callable,` `Comparator,` or Java 8’s new interfaces, such as `Function,` `Predicate,` and `Consumer.`
Lambdas are most often used in collection operations (e.g., filtering, mapping, and sorting) using the Stream API. They are also heavily used for event handling (e.g., action listeners in GUIs) and asynchronous programming. They make Java code much more readable and maintainable by providing a compact way of expressing behavior without full class definitions or anonymous inner classes.
Java 8 Stream API Coding Questions for Experienced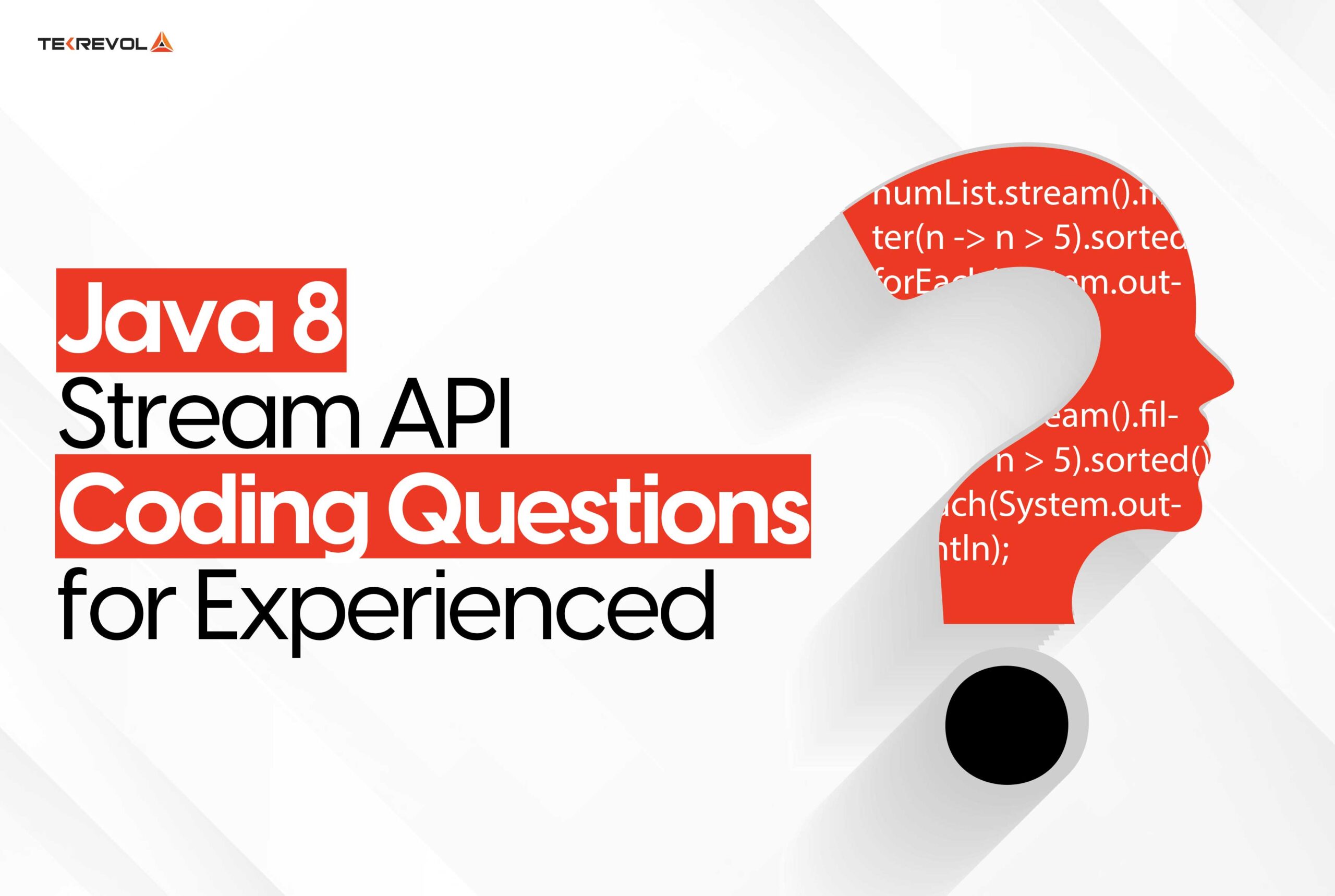
26.How do you filter data using the Stream API?
Filtration is a core concept and is sure to be asked in Java 8 features interview questions!
The `filter` method of the Stream API filters elements from a collection according to some condition (predicate). It creates a new stream containing only the elements that satisfy the condition. This is especially helpful if you need to process only a subset of data.
For instance, you might have a collection of numbers and want to get rid of all even numbers. You can use the `filter` collector with a predicate that checks whether each number is odd. The original collection is not modified, thereby staying immutable and safe when working with streams.
27.What is the purpose of `collect` in Streams?
The `collect` method is another terminal operation in the Stream API that transforms a stream’s elements into another form, such as a list, set, or map. It collects results after processing the stream pipeline.
For example, if you are processing a stream of integers and want to collect only the even numbers into a list, then you can use `collect` with `Collectors.toList()`. This has a wide range of applications, and you can actually implement your custom collector to suit complex demands such as grouping or partition based on some conditions.
While preparing for Java 8 features interview questions, this is one of the core concepts that you should know!
28.Does Streams handle infinite data?
Yes, you can handle infinite data using a Stream API. For that, you can use methods like `Stream. Generate` and `Stream. Iterate`. These allow you to produce an endless stream of elements. Since an infinite stream cannot be fully processed, you must include some operations like `limit` to work with a finite subset of the data.
For instance, while using `Stream.iterate(1, n -> n + 1)`, you can create an infinite sequence of numbers. Then, you can limit the result to only the first 10 numbers. Streams with lazy evaluation only process the elements that are necessary for the given operation – to make the code look efficient.
29.How do you sort a stream?
You can sort a stream using the sorted method, which is an intermediate operation; by default, it sorts elements in their natural order-for example, ascending for numbers. If you want to sort in a custom order, you can pass a comparator to the sorted method.
For example, suppose you have a list of employees and wish to sort them by salary in descending order can supply a comparator that compares the salaries in reverse. Sorting with Streams is immutable: the original collection is not modified; instead, its results are stored in a new stream.
Sorting is something that you would do every day while handling projects, which makes it important for you to remember the answer to this Java 8 features interview question.
30.What is the role of `reduce` in Streams?
The `reduce` method in Stream is used for aggregation operations on a stream’s elements, collecting them into a single result. The argument would be a binary operator – or an identity value that comes together with a binary operator. It can be used to sum numbers, concatenate strings, or calculate the product of a list of integers, among other applications.
For instance, to reduce a list of numbers into the sum, you would use `reduce(0, Integer::sum)`. The element acts as the starting point, and the binary operator gives the way to combine two elements at each step of the reduction process.
- Why settle for good when you can be exceptional?
- Gain access to top-tier resources, training, and opportunities With Tekrevol.
- Enjoy Your Free Consultation Now
Scenario-Based Java 8 Interview Questions
31.How would you merge two lists using Streams?
While preparing for Java 8 features interview questions, you can be asked about merging two lists using streams. This can easily be done using the “Stream.concat” method. It merges two streams into one that you can collect into a list through a terminal operation like `collect.`
Here’s how it works:
You create a stream from both lists using `Stream.concat` to concatenate them and collect the merged stream back into a new list. For example, you may have two lists of integers. After the merge, duplicates will remain unless you further apply a method like `distinct.` This approach is concise, thread-safe, and avoids manual iteration through elements, which makes your code cleaner and more maintainable.
32.How would you use Java 8 to find the first non-repeated character in a string?
It won’t be wrong to say that this is the toughest Java 8 features interview question, one that can shake the confidence of even experienced candidates.
To get the first non-repeated character in a string using Java 8’s Stream API, you can create a stream of characters by converting the string to an array or list and then map each character to its frequency by using `Collectors.groupingBy`.
Finally, you filter the entries to collect those characters with a count of one and pick up the first one. For instance, on the string `\”swiss\,”` after creating the frequency map, the stream decides `’w’` as the first unique character. This can easily be done by using nested loops. Thus, this technique is more readable and also faster for large strings.
33.How would you count duplicate elements in a list?
To count duplicate elements in a list using Java 8, leverage the Collectors.groupingBy and Collectors.counting methods. First, create a stream from the list, then use `groupingBy` to group elements by their values. Inside the collector, apply `counting` to compute the frequency of each element.
Finally, filter the map entries to extract elements with a count greater than one, indicating duplicates. For instance, groupingBy will return 1=1, 2=2, 3 =3 From a list like [1, 2, 3, 3, 3], showing 2 and 3 appear there as duplicates in the list.
34.How would you convert a list to a map using Streams?
If you want to convert a list of values into a map in Java 8, you can easily do this using the Collectors.toMap method. Using lambda expressions, each element in the list can be transformed into a key-value pair.
Here’s how you’d do it if you wanted a list of strings where the key could be the string itself and the value could be the string’s length: Use `Collectors.toMap` to transform the list into a map. If there are duplicate keys, you have to provide a merge function to handle the conflict. This is also very useful if you want to set any logic for keys and values; thus, it fits a lot of mapping needs.
If you only answered stream-related queries confidently in Java 8 features interview questions, we can assure you that no one can stop you from getting the job.
35.How do I eliminate null checks in a method using Optional?
The Optional class of Java 8 was designed to minimize null pointer exceptions by explicitly handling the values optionally.
Instead of returning `null,` a method can return an `Optional` object. You can avoid checking for nulls using a chain of methods that include `isPresent` or `ifPresent` methods to check if a value exists or to execute some code if it’s already present.
With `orElse,` you can specify a default value, and with `orElseGet,` a fallback supplier. So, if you have a method that returns an `Optional,` you could write something like `userOptional.ifPresent(user -> process(user))`. This makes your code cleaner and more robust.
Java 8 Coding Interview Questions
36.How do you reverse a list using Streams?
Reversing is the most commonly asked Java 8 Interview question.
To reverse a list in Java 8, you can use Streams with a sort and a custom comparator or by using the Collections.reverseOrder() method. You first convert your list into a stream, then process it in reverse order, and finally, collect the result into a new list.
Alternatively, you could convert the stream into an array, reverse the array indices, and collect it back into a list. The key benefit to using Streams is that the process is more declarative or more concise compared to traditional loops. This is a very commonly asked question to test your understanding of how Streams simplify complex operations.
37.How would you group data in a collection by a field?
Using the `Collectors.groupingBy()` method in Java 8, grouping data by a field is very easy.
This powerful collector groups elements in a stream based on a classifier function, like a field in an object. For instance, if you have a collection of employees and would like to collect them by department, the classifier for this purpose may be `getDepartment().`
The result would be a `Map,` where keys are names of different departments, and values are lists of employees in each department. This is a very common pattern in data aggregation operations and shows how functional programming makes operations like this much more straightforward.
38.How do you find the maximum value in a stream?
Apart from reversing the string, the most common Java 8 interview\questions revolves around finding the maximum and minimum value in a stream.
To find the maximum value in a stream, Java 8 provides the `max()` terminal operation, which takes a comparator as an argument.
For example, if you have a stream of integers, you can use `Comparator.naturalOrder()` to find the largest value.
Similarly, for custom objects such as employees, you may pass a comparator with a specific column, such as salary or age. The `max()` operation returns an `Optional,` which you can handle using methods like `orElse()` or `ifPresent().` This approach lets you eliminate the need for manual comparisons, which is a fantastic demonstration of how Java 8 reduces boilerplate code.
39.How do you remove duplicates from a list using Streams?
Java 8’s `distinct()` method eliminates duplicate elements from a list. Applied to a stream, `distinct()` filters such that elements in the resulting stream are encountered for the first time. For instance, from the list of integers that may contain duplicates, converting to a stream and using the `distinct()` method will return a stream of unique values only.
The final result can be collected into a new list with `Collectors.toList()`. This is a simple, effective way to operate on collection objects without touching the original list, and it is an example of how Java 8 supports immutable operations and thread safety.
49.How do you sort a map by its values in Java 8?
In Java 8, sorting a map based on its values is done by converting the map’s entry set into a stream, sorting the entries based on their values, and finally collecting the result into a new map.
The `sorted()` method is applied with a comparator that compares the values of the map entries. Then, the `Collectors.toMap()` method can rebuild the sorted map. This method is useful for big datasets since it can reorder a map cleanly and functionally. This is a very common interview question that tests your aptitude when handling complex data manipulation.
Why Choose Tekrevol?
If you’re still reading this, then the chances are high that you are now aware of most of the Java 8 interview questions—but if there’s something more, we’re here to help!
Tekrevol isn’t just another Mobile App Development Company in San Francisco—it’s a community of innovators dedicated to helping individuals and businesses unlock their full potential.
Our team has built cutting-edge solutions using Java 8 and beyond, and we’re committed to passing that expertise on to you. From curated training resources to hands-on workshops, Tekrevol is where you’ll find opportunities to grow.
Whether you’re preparing for an interview or building enterprise-grade applications, Tekrevol has the tools and mentors to guide your journey. With Tekrevol, choose success backed by a proven track record of innovation and excellence.
Let’s build the future together!
Share Your Feedback